Arrays in C Programming: In C programming, arrays are a fundamental data structure that allows you to store a fixed-size sequential collection of elements of the same type. Arrays are particularly useful when you need to work with multiple data items of the same kind, such as a list of integers, characters, or any other data type.
C Programming Video Tutorial Lecture-07
Table of Content
What is Arrays in C Programming?
An array is a collection of elements, each identified by an array index. The array is stored in contiguous memory locations, meaning all the elements are stored next to each other in memory.
Declaration of Arrays
type arrayName[arraySize];
type: The data type of the elements (e.g., int, float, char).
arrayName: The name of the array.
arraySize: The number of elements the array can hold.
Example:
int numbers[10];
This creates an array named numbers that can hold 10 integers.
Initialization of Arrays
You can initialize an array at the time of declaration by specifying the values in curly braces.
Example:
int numbers[6] = {100, 200, 300, 400, 500,600};
You can also partially initialize an array:
int numbers[5] = {4, 10}; // Remaining elements are initialized to 0.
Or leave it uninitialized:
int numbers[5]; // Contains garbage values until initialized.
Accessing Array Elements
Array elements are accessed using the index, with the first element at index 0.
Example:
printf(“%d”, numbers[0]); // Outputs 4
Modifying Array Elements
You can modify elements by assigning a new value to a specific index.
Example:
numbers[2] = 8; // Changes the third element to 8
Types of Arrays in C Programming
One-Dimensional Arrays
A simple array with a single row of elements.
Example:
char vowels[7] = {‘z’,’e’, ‘e’, ‘s’, ‘h’, ‘a’, ‘n’};
Multi-Dimensional Arrays
An array of arrays. The most common form is the two-dimensional array, often used to represent matrices.
Example:
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
In this example, matrix is a 3×3 array.
Character Arrays (Strings)
A special type of array used to store sequences of characters.
Example:
char name[10] = “John”;
The string “John” is stored in a character array with space for 10 characters.
Passing Arrays to Functions in C Programming
In C, you can pass arrays to functions. When you pass an array to a function, you’re actually passing the address of the first element of the array.
Example:
void printArray(int arr[], int size) {
for(int i = 0; i < size; i++) {
printf(“%d “, arr[i]);
}
}
Common Operations on Arrays in C Programming
- Traversal: Iterating through each element.
- Searching: Finding a specific element (e.g., linear search, binary search).
- Sorting: Arranging elements in a certain order (e.g., bubble sort, quicksort).
- Merging: Combining two arrays into one.
Advantages of Using Arrays in C Programming
- Fast Access: Direct access to elements using indices.
- Memory Efficiency: Arrays are efficient in terms of memory usage as they are stored in contiguous blocks of memory.
Disadvantages of Arrays in C Programming
- Fixed Size: Once declared, the size of an array cannot be changed.
- Memory Wastage: If the array size is larger than needed, memory is wasted.
Common Mistakes with Arrays in C Programming
- Index Out of Bound: Accessing an index that doesn’t exist, leading to undefined behavior.
- Uninitialized Arrays: Failing to initialize arrays can result in unpredictable values.
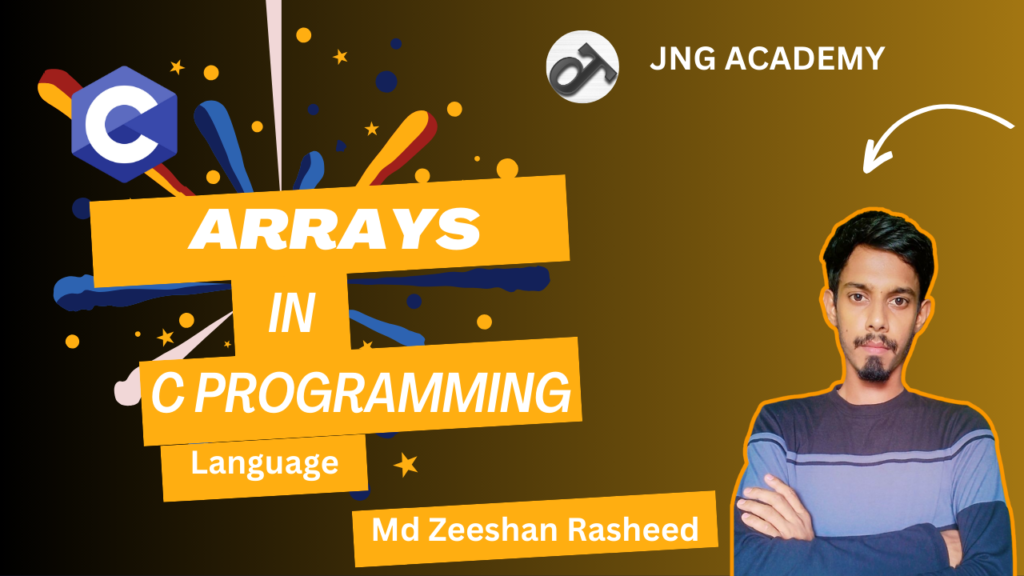
Frequently Asked Questions (FAQs)
What is an array in C?
How do I declare an array in C?
Can I change the size of an array after it’s declared?
How are arrays stored in memory?
What happens if I access an array element outside its bounds?
How do I initialize an array in C?
Can I pass an array to a function in C?
What is a multi-dimensional array?
How do I determine the size of an array in C?
What is the difference between an array and a pointer?
Learn More
Subscribe “JNG ACADEMY” for more learning best content.
Important Links
C Programming Language Lecture-01
C Programming Language Lecture-02
C Programming Language Lecture-03
C Programming Language Lecture-04
C Programming Language Lecture-05
C Programming Language Lecture-06
C Programming Langauge Lecture-07
C Programming Language Youtube Playlist
Thank You So Much, Guys… For Visiting our Website and Youtube.