Static and Dynamic Scoping in C Programming: When writing code, especially in C and other programming languages, how variables are accessed and resolved is an essential aspect of scope management. Two fundamental approaches to determining the scope of variables are static scoping and dynamic scoping. In this article, we will explore both scoping mechanisms, their differences, how they work, and where they are used.
Pointers in C Programming Language Link
What is Scoping in Static and Dynamic Scoping in C Programming?
Scope defines the region of a program where a particular variable or function can be accessed. There are two major scoping rules used in programming languages:
- Static Scoping (Lexical Scoping)
- Dynamic Scoping
While C uses static scoping, understanding dynamic scoping is important to grasp how some other languages work and for comparative study.
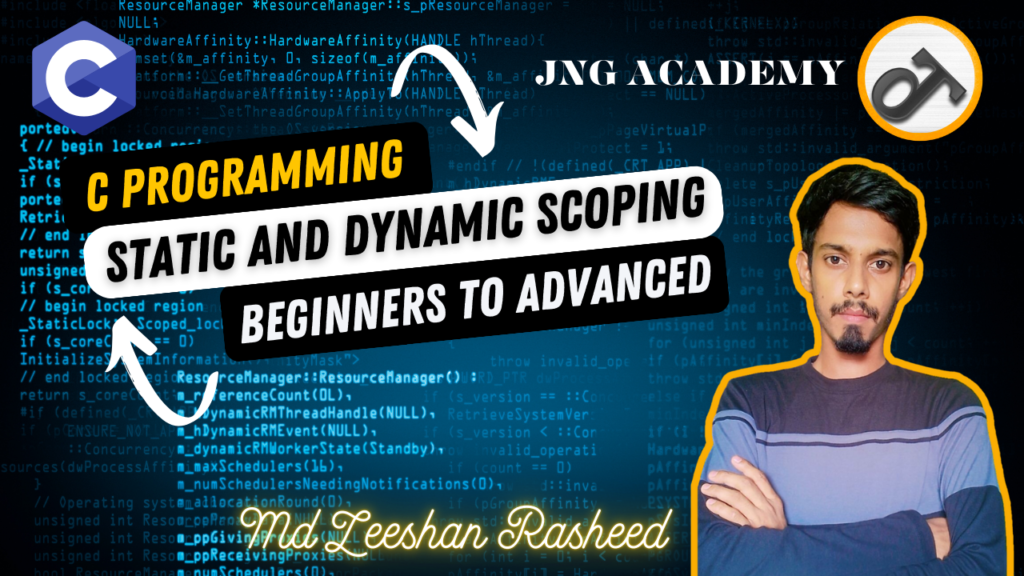
Static and Dynamic Scoping in C Programming
Static scoping, also called lexical scoping, determines the scope of a variable at compile time. It is the most commonly used scoping rule in many programming languages, including C, C++, Java, and Python.
How Static Scoping Works:
In static scoping, a variable’s scope is determined by the structure of the code. The compiler decides which variable to reference based on where it is declared in the source code. In other words, the location in the code where a variable is defined dictates its visibility.
Example of Static Scoping:
#include<stdio.h>
int x = 10; // Global variable with file scope
void func() {
printf(“%d\n”, x); // Refers to the global x
}
int main() {
int x = 20; // Local variable with block scope
func(); // This will print 10 because func() uses the global x
return 0;
}
In the above example, the func() function uses the global variable x because its scope is determined at compile time. The local x defined inside main() does not affect func().
Key Features of Static Scoping:
- Determined at compile time: The compiler resolves variable references by looking at the source code.
- Predictable: You can trace the scope of a variable by looking at the program’s code structure.
- Nested Scopes: A block of code can access variables from its enclosing blocks (parent blocks), but it cannot access variables from inner blocks.
Advantages of Static Scoping:
- Easier to understand and maintain: Since the scope of each variable is clear and fixed, code is easier to debug and reason about.
- Faster Execution: The compiler resolves variable references during compilation, making the execution faster since there’s no need for runtime resolution.
Dynamic Scoping in C Programming
Dynamic scoping is a less commonly used method of variable resolution. In this type of scoping, the scope of a variable is determined at runtime, based on the sequence of function calls. Unlike static scoping, the variable that gets accessed depends on the call chain that leads to a particular function.
How Dynamic Scoping Works:
In dynamic scoping, when a variable is used, the runtime system looks up the call stack to find the most recent value of that variable in the function calls. If a variable is declared in multiple functions along the call chain, the closest one in terms of the calling order is used.
Example of Dynamic Scoping (Hypothetical):
Let’s consider how dynamic scoping would work, even though C does not support it.
int x = 1;
void func1() {
printf(“%d\n”, x);
}
void func2() {
int x = 2;
func1();
}
int main() {
int x = 3;
func2(); // Under dynamic scoping, func1() would print 2
return 0;
}
In this example, if C used dynamic scoping, func1() would print 2 because func2() redefines x before calling func1(). However, in static scoping (which C uses), func1() would print 1, as it uses the global x.
Key Features of Dynamic Scoping:
- Determined at runtime: The interpreter resolves variable references by looking at the runtime call stack.
- Unpredictable: The value of a variable depends on the sequence of function calls, not the program’s code structure.
- No Nested Scopes: A function can access variables from its calling functions, regardless of the lexical structure of the code.
Example in a Language with Dynamic Scoping (e.g., Lisp):
Languages like Emacs Lisp and Bash use dynamic scoping. In Emacs Lisp:
(setq x 10)
(defun func1 ()
(print x)) ;; This function prints the value of x
(defun func2 ()
(let ((x 20)) ;; Dynamically scoped variable x
(func1)))
(func2) ;; Prints 20 because func2 dynamically scopes x
Advantages of Dynamic Scoping:
- Flexibility: It allows functions to adapt based on the current environment of function calls.
- Useful in certain contexts: Dynamic scoping can be useful in environments where you need functions to inherit variables from calling functions automatically.
Disadvantages of Dynamic Scoping:
- Harder to debug: Since variable references are resolved at runtime, tracking down bugs or unintended behavior can be difficult.
- Performance hit: Variable resolution must be done at runtime, which can slow down the execution of programs.
- Unpredictable behavior: Variables can take on values that are hard to anticipate based on the code, leading to less maintainable code.
Differences Between Static and Dynamic Scoping in C Programming
Feature | Static Scoping | Dynamic Scoping |
---|---|---|
Resolution | Compile-time | Runtime |
Variable Reference | Based on the structure of the code | Based on the call chain of functions |
Predictability | Highly predictable, based on the code | Less predictable, depends on call sequence |
Access | Enclosing Blocks or global scope | Closest scope in the call stack |
Use Cases | Used in C, C++, Java, Python etc. | Used in Lisp, Emacs Lisp, Bash etc. |
Which One Should You Use Static and Dynamic Scoping in C Programming?
Static Scoping is the default in most modern programming languages because of its predictability and ease of debugging.
Dynamic Scoping is used in specific languages and situations where more flexibility in accessing variables is required. It can be useful for certain use cases in scripting languages or environments where functions need to inherit variables from their callers.
Summary
Understanding the differences between static and dynamic scoping is essential for grasping how variables are handled in different programming languages. While C and most modern languages use static scoping, dynamic scoping can still be encountered in certain programming environments. By knowing the strengths and weaknesses of both scoping mechanisms, you can better structure your code and select the right approach for your specific needs.
Frequently Asked Questions (FAQs)
What is static scoping in programming?
What is dynamic scoping?
Which programming languages use static scoping?
What are examples of languages that use dynamic scoping?
Can a language use both static and dynamic scoping?
Why is static scoping preferred over dynamic scoping in most languages?
What are the advantages of dynamic scoping?
What are the disadvantages of dynamic scoping?
How does C handle scoping?
Can I switch between static and dynamic scoping in C?
What is variable shadowing in static scoping?
How do I avoid scope-related bugs in static scoping?
Use descriptive and unique variable names for global and local variables.
Keep global variables to a minimum and prefer local variables whenever possible.
Learn More
Subscribe “JNG ACADEMY” for more learning best content.
Important Links
C Programming Language Lecture-01
C Programming Language Lecture-02
C Programming Language Lecture-03
C Programming Language Lecture-04
C Programming Language Lecture-05
C Programming Language Lecture-06
C Programming Language Lecture-07
C Programming Langauge Lecture-08
Thank You So Much, Guys… For Visiting our Website and Youtube.