Functions in C Programming: C programming is one of the most powerful and flexible languages available. To harness its full potential, mastering functions is a must. Functions are the cornerstone of structured programming in C, allowing for better modularity, readability, and reusability. In this comprehensive guide, we’ll dive deep into the world of functions in C, exploring everything from basic definitions to advanced concepts like recursion and function pointers.
C Programming Video Lecture-05
Table of Contents
1. Introduction to Functions in C Programming
1.1 What is a Function?
In C programming, a function is a self-contained module of code that accomplishes a specific task. Think of functions as the basic building blocks of your program. Just like bricks in a building, functions can be combined in various ways to create complex programs.
The primary benefits of using functions include:
- Modularity: Breaking down a large program into smaller, manageable parts.
- Reusability: Once written, a function can be reused in different parts of a program or even in different programs.
- Ease of Maintenance: Functions help keep your code organized, making it easier to understand, debug, and maintain.
1.2 Types of Functions
Functions in C can be broadly classified into two categories:
Library Functions: Predefined functions that are part of the C standard library, such as printf(), scanf(), strlen(), etc. These functions are already compiled, so you don’t need to write the code for them—just include the appropriate header files (e.g., stdio.h, math.h) in your program.
User-Defined Functions: Functions created by the programmer to perform specific tasks. These functions are defined and implemented according to the needs of your program.
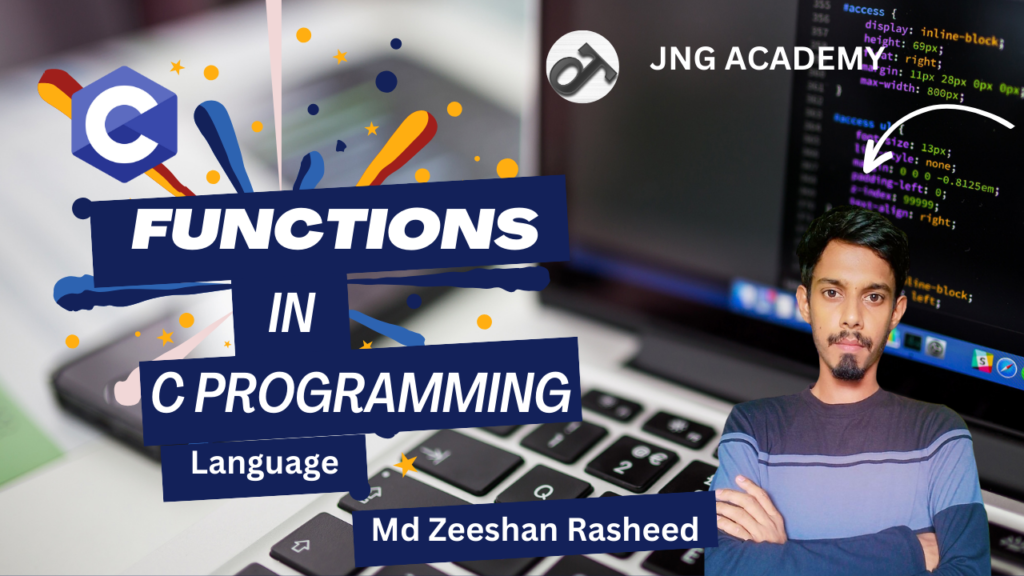
2. Anatomy of a C Functions in C Programming
Understanding the structure of a function is crucial for writing effective code. A typical function in C consists of the following components:
2.1 Function Return Type
The return type specifies the data type of the value that the function will return to the calling function. Common return types include:
- int: Returns an integer value.
- float: Returns a floating-point value.
- char: Returns a character.
- void: Indicates that the function does not return a value.
2.2 Function Name
The function name is a unique identifier for the function, following the same naming conventions as variables. It should be descriptive and meaningful, reflecting the function’s purpose.
2.3 Parameters (Arguments)
Parameters are variables that the function takes as input. These are enclosed in parentheses and separated by commas. If the function doesn’t require any input, the parentheses are left empty.
- Formal Parameters: The variables defined in the function declaration that receive the values passed to the function.
- Actual Parameters: The actual values passed to the function when it is called.
2.4 Function Body
The function body is the block of code enclosed in curly braces {} that defines what the function does. This is where you write the code that will be executed when the function is called.
2.5 Return Statement
The return statement is used to exit a function and optionally return a value to the calling function. The data type of the returned value must match the function’s return type.
Example:
int add(int a, int b) {
int sum = a + b;
return sum;
}
In this example, add is a function that takes two integer parameters and returns their sum.
3. Declaration, Definition, and Call Functions in C Programming
3.1 Function Declaration
A function declaration, also known as a function prototype, tells the compiler about the function name, return type, and parameters. This helps the compiler to correctly interpret function calls. The declaration is typically placed at the beginning of the program, before the main() function.
return_type function_name(parameter_list);
Example:
int add(int, int);
3.2 Function Definition
The function definition provides the actual implementation of the function. This is where you write the code that will be executed when the function is called.
Syntax:
return_type function_name(parameter_list) {
// function body
}
Example:
int add(int a, int b) {
int sum = a + b;
return sum;
}
3.3 Function Call
A function call is an instruction that tells the program to execute the code within a function. When the function is called, control is transferred to the function’s code block.
Syntax:
function_name(arguments);
Example:
int result = add(5, 3);
In this example, the add function is called with 5 and 3 as arguments, and the result is stored in the variable result.
4. The main() Functions in C Programming
The main() function is the entry point of every C program. When a C program is executed, the control starts from the main() function. It can be written as:
int main() {
// Your code here
return 0;
}
Here, return 0; indicates that the program has terminated successfully. The main() function can also accept command-line arguments, which are passed to the program when it is executed.
Example:
int main(int argc, char *argv[]) {
printf(“Hello, World!\n”);
return 0;
}
In this example, argc is the number of command-line arguments, and argv is an array of strings representing the arguments.
5. Arguments and Parameters Functions in C Programming
5.1 Pass by Value
In C, when arguments are passed to a function, they are typically passed by value. This means that the function receives a copy of the argument’s value. Changes made to the parameter within the function do not affect the original argument.
Example:
void modifyValue(int x) {
x = 10;
}
int main() {
int a = 5;
modifyValue(a);
printf(“%d”, a); // Output: 5
return 0;
}
In this example, the value of a remains unchanged because modifyValue() only modifies a copy of a.
5.2 Pass by Reference
To allow a function to modify the original value of an argument, you need to pass the argument by reference. In C, this is done using pointers.
Example:
void modifyValue(int *x) {
*x = 10;
}
int main() {
int a = 5;
modifyValue(&a);
printf(“%d”, a); // Output: 10
return 0;
}
In this example, the value of a is modified because modifyValue() receives a reference to a rather than a copy.
6. Return Statement Functions in C Programming
The return statement is used to exit a function and optionally return a value to the calling function. If the function has a non-void return type, the return statement must be followed by a value of that type.
6.1 Returning Values
A function can return a value to the caller using the return statement. The value returned must match the function’s declared return type.
int multiply(int a, int b) {
return a * b;
}
int main() {
int result = multiply(4, 5);
printf(“%d”, result); // Output: 20
return 0;
}
6.2 Returning Multiple Values
C does not allow a function to return multiple values directly. However, this can be achieved by returning a structure, using pointers, or passing arguments by reference.
Example using Structures:
struct Pair {
int first;
int second;
};
struct Pair getPair() {
struct Pair p;
p.first = 1;
p.second = 2;
return p;
}
int main() {
struct Pair myPair = getPair();
printf(“First: %d, Second: %d”, myPair.first, myPair.second); // Output: First: 1, Second: 2
return 0;
}
7. Recursive Functions in C Programming
Recursion is a technique where a function calls itself. Recursive functions are useful for solving problems that can be broken down into smaller, similar problems. However, recursion must be used with caution to avoid infinite loops and stack overflow errors.
7.1 Basic Example of Recursion
A classic example of recursion is calculating the factorial of a number.
Example:
int factorial(int n) {
if (n == 0)
return 1;
else
return n * factorial(n – 1);
}
int main() {
int result = factorial(5);
printf(“%d”, result); // Output: 120
return 0;
}
7.2 Recursive vs. Iterative Solutions Functions in C Programming
Aspect | Recursive Solution | Iterative Solution |
---|---|---|
Approach | Function calls itself to solve smaller subproblems. | Uses loops to repeatedly execute a block of code. |
Memory Usage | Higher memory usage due to multiple function calls on the stack. | Lower memory usage as it operates within as single stack frame. |
Risk of Stack overflow | High, expecially with deep recursion or large inputs. | Low, as it doesn’t depend on the call stack for repetion. |
Performance | Generally slower due to the overhead of function calls. | Typically faster and more efficient, especially for large inputs. |
Simplicity & Readability | More elegant for problems naturally defined by subproblems. | Often more straightforward for problems with clear iterations. |
Use case | Ideal for problems like tree/graph traversal, and divid-and-conquer algorithm (e.g., QuickSort, MergeSort). | Preferred for tasks like array manipulation, searching, and simple mathematical operations. |
Code Length | Often shorter and more concise. | May requires more lines of code, especially for complex problems. |
Base Case Rquirement | Requries a base case to prevent infinite recursion. | No base case needed; loop conditions control termination. |
Frequently Asked Questions (FAQs)
What are functions in C programming?
What is the difference between main() and other functions in C?
How can I pass parameters to a function in C?
What is recursion in C?
What is the difference between recursion and iteration?
Can a C program run without a main() function?
main()
function is essential because it serves as the entry point for program execution. Without it, the program cannot run.What are pointers in C?
Learn More
Subscribe “JNG ACADEMY” for more learning with best content.
Important Links
C Programming Tutorial Lecture-01
C Programming Tutorial Lecture-02
C Programming Tutorial Lecture-03
C Programming Tutorial Lecture-04
C Programming Tutorial Lecture-05
C Programming Tutorial Playlist
Thank You So Much, Guys… For Visiting our Website and YouTube.